Solidity Smart Contract Template
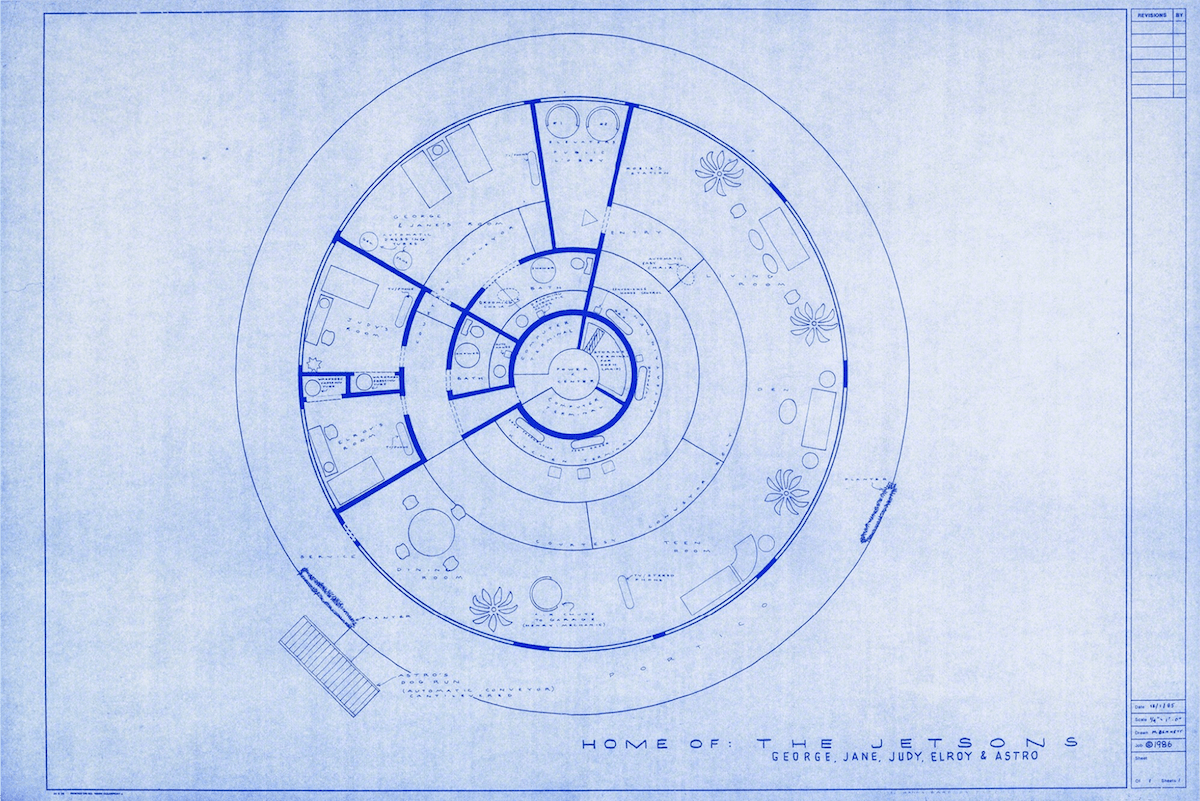
When you start writing smart contracts that are a step or two beyond simplistic, tutorial-level code, you quickly have more code than can easily fit on a screen, and with each new function or added variable, there's more and more need for structure and for easy way to know exactly what to put where, and how.
Importance of a proper contract layout
- Readability: Makes the code understandable for developers and auditors.
- Maintainability: Facilitates easy updates and bug fixes, both by you and by whoever uses your code.
- Efficiency: Properly structured contracts can lead to optimized gas usage.
- Security: Reduces the risk of vulnerabilities due to code complexity or ambiguity.
- Reusability: Makes it easy to reuse certain components or modules in different projects.
- Education: Good-quality code teaches best practices whoever reads the code you have written.
What you need to consider
When writing smart contracts in Solidity, you need to take many things into account. Here are just a few:
- Style guide - general conventions for writing Solidity code, changing over time as useful conventions are found and old conventions are rendered obsolete
- Solidity file elements - what goes where in a Solidity file
- Contract elements - the order of elements inside a contract, an interface, or a library
- Function grouping - the order of functions based on their types
- Naming convention - how to name functions, variables, contracts so that it's easier to see what they do and where they belong
Solidity contract starter template
To make it easier, so that you don't need to remember everything, I have created a Solidity contract starter template, with all the best practices, conventions, recommendations, good layout, and proper structure.
You can reference it as a cheatsheet or use it as a better alternative for an empty file. In it, you'll find all the placeholders telling you what goes where. You'll only need to fill it with your code.
Because style guides change and the Solidity language itself also changes, the conventions are not set in stone, so I'm creating this template also as a GitHub repository, where you can add issues and create PRs. Let's build the best starter template, together.
Solidity smart contract template with explanations
Here you can find the code in GitHub: Solidity Smart Contract Template repository - GitHub You can go ahead and give it a ⭐.
You can refer to this template as a cheatsheet or use it as a better alternative for an empty file. In it, you'll find all the placeholders telling you what goes where.
The only thing left to do is to fill it with your code.